在我们写代码的过程中,出现错误是非常常见的事情,如何对一些异常进行合理方式的处理是非常重要的问题,c语言有c语言的方法,当然,cpp作为oop的语言当然也有它的一套体系。
C语言处理错误的方式
c错误处理方式
-
终止程序:比如assert,缺点:有些问题会直接退出会让用户非常难受,比如出现网络错误,我们通常希望的是告警,而不是进程退出。
-
返回错误码:返回错误码的方式并不直观,比如返回一个5,并不能直接知道出了什么错误,必须要查对应的的错误。
大部分情况c还是采用返回错误码的方式处理错误,部分情况使用终止进程处理特别严重的错误。
C++异常概念
异常是什么?其实是一种处理错误的方式,当一个函数遇到无法处理的错误时,就可以抛出异常,让函数可以直接或者间接的调用者去处理这个错误。
大概形式如下:
1 2 3 4 5 6 7 8 9 10 11 12 13
| try { }catch( ExceptionName e1 ) { }catch( ExceptionName e2 ) { }catch( ExceptionName eN ) { }
|
异常的使用
关键字
使用异常前,先认识一下异常的关键字
- throw:抛出异常关键字,可以抛出任意的东西(字符串、整数…)
- try:括号内的代码就是可能会出现异常的地方。
- catch:能捕捉抛出的异常,当然要类型对应。
异常规则
异常的抛出和捕获
- 抛出对象的类型严格匹配catch类型
- 抛出对象匹配最近的catch
- 抛出的是对象的拷贝
- catch(…) 可以捕捉任意类型的异常,用来兜底
- 可以抛派生类对象,用父类进行捕获
类型匹配例子:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| void fun() { int a, b; cin >> a >> b; if (b == 0) { throw 'e'; } else { cout << "a/b=" << a / b << endl; } }
int main() { try { fun(); } catch (int errid) { cout << "errid:" << errid << endl; } catch (char errch) { cout << "errch:" << errch << endl;
} catch (const string& errstr) { cout << "errstr:" << errstr << endl; }
return 0; }
|
运行结果如下,匹配对应的catch
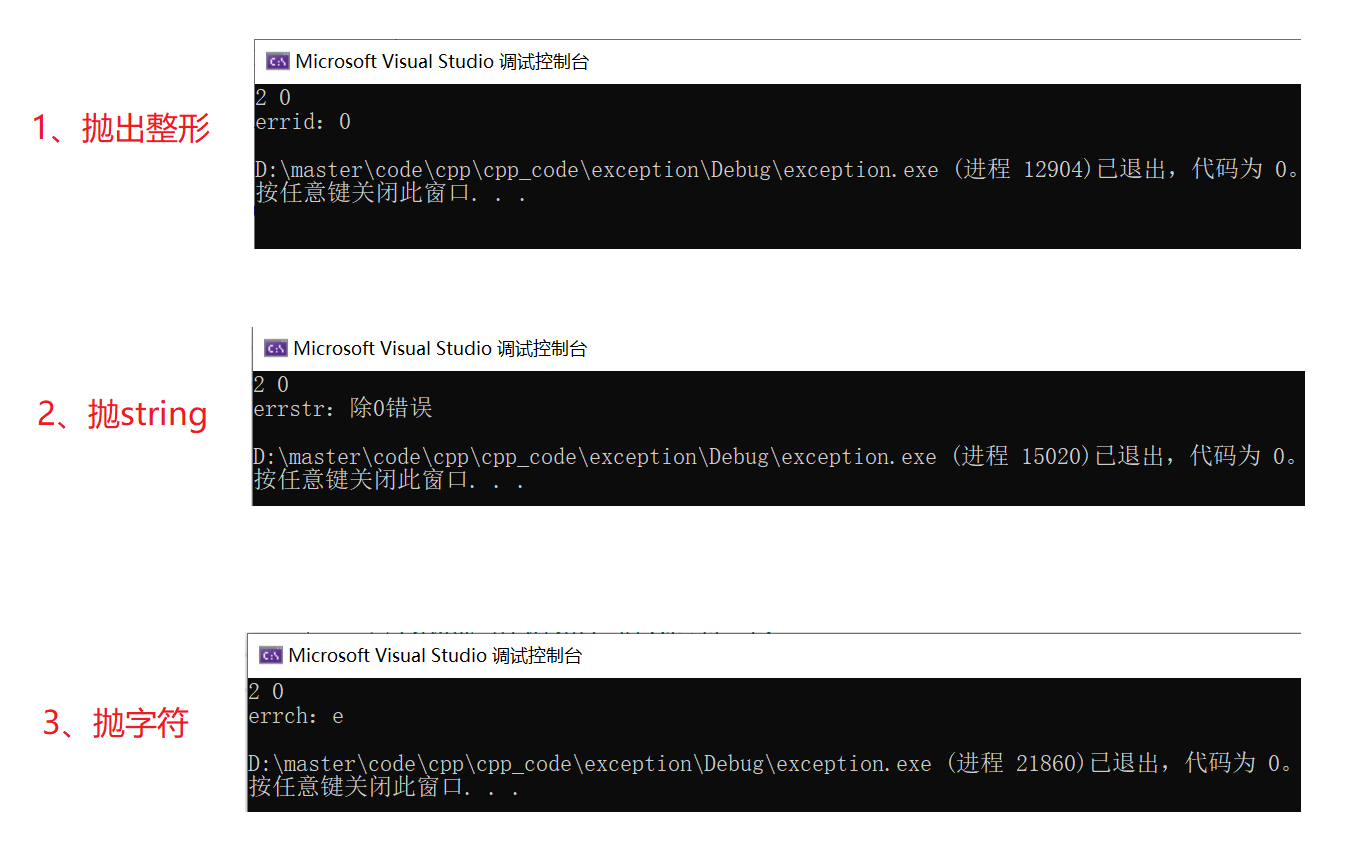
子类异常基类捕获
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| class Exception { public: Exception(const string& errmsg, int id) :_errmsg(errmsg) , _id(id) {} virtual string what() const { return _errmsg; } int getid() const { return _id; }
protected: string _errmsg; int _id; };
class ErrorA : public Exception { public: ErrorA(const string& errmsg, int id, const string& errorA) :Exception(errmsg,id) ,_errA(errorA) { } virtual string what()const { string str = _errmsg + " " + _errA; return str; } private: string _errA; };
void fun() { srand(time(nullptr)); if (rand() % 5 == 0) { throw ErrorA("错误:", 100, "A"); } } int main() { while (1) { Sleep(1000); try { fun(); } catch (const Exception& e) { cout << e.what() << endl; } catch (...) { cout << "未知错误" << endl; } }
return 0; }
|
运行结果:
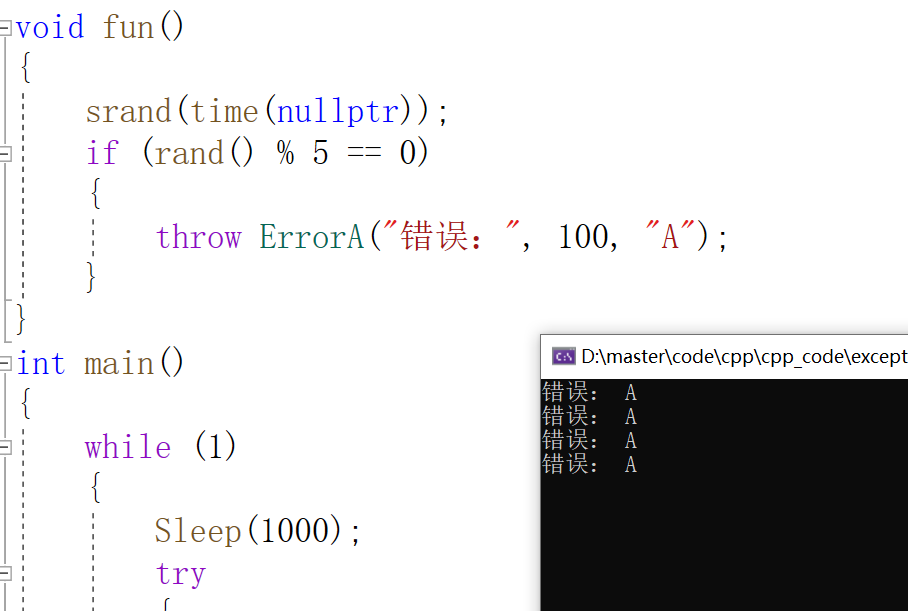
在函数调用链中异常展开匹配规则
- 现在当前栈帧中看
- 没有去上一层栈帧
- 如果到了main栈帧还未匹配,终止程序
- catch处理后,会继续执行之后的语句
函数调用例子
代码大致如上方代码,但是fun函数里面调用了div函数,并在fun函数里面进行异常捕获,同样的在main函数中,也对fun进行异常的捕获
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106
| void div() { int a, b; cin >> a >> b; if (b == 0) { throw string("除0错误"); } else { cout << "a/b=" << a / b << endl; } }
void fun() { try { div(); } catch (int errid) { cout << "fun中catch:" << errid << endl; } }```
运行结果:
 {
int* array1 = new int[10]; int* array2 = new int[10];
int len, time; cin >> len >> time;
try { cout << Division(len, time) << endl; } catch (...) { cout << "delete []" << array1 << endl; delete[] array1;
cout << "delete []" << array1 << endl; delete[] array2; throw; }
cout << "delete []" << array1 << endl; delete[] array1;
cout << "delete []" << array2 << endl; delete[] array2; }
int main() { try { Func(); } catch (const char* errmsg) { cout << errmsg << endl; }
return 0; }
|
异常规范
- 异常规格说明的目的是为了让函数使用者知道该函数可能抛出的异常有哪些。 可以在函数的后面接throw(类型),列出这个函数可能抛掷的所有异常类型。
- 函数的后面接throw(),表示函数不抛异常。
- 若无异常接口声明,则此函数可以抛掷任何类型的异常
1 2 3 4 5 6 7 8 9 10 11 12
| void fun() throw(A,B,C,D);
void* operator new (std::size_t size) throw (std::bad_alloc);
void* operator delete (std::size_t size, void* ptr) throw();
thread() noexcept; thread (thread&& x) noexcept;
|
异常的优缺点
C++异常优点
- 异常对象定义好之后,相比错误码的方式更能清晰准确的展示出错误的各种信息,甚至可以包含调用堆栈的信息,可以更容易定位程序bug
- 可以直接跳出到catch捕捉的地方,而不用像错误码一样层层返回。
- 很多第三方库包含异常
- 部分函数使用异常更好处理,比如构造函数没有返回值,不方便使用错误码方式处理,方括号的重载,pos越界错误,只能通过异常或者终止程序。
C++异常缺点
- 执行流可能会乱跳,运行时抛出,会比较的混乱
- 有性能开销
- 容易导致内存泄漏、死锁安全问题
- C++标准体系定义不好,导致大家自定义各自的异常体系,十分混乱
总结
异常尽量规范使用,不要随便抛异常,遵守如下:
- 所有异常类型都继承于一个基类
- 函数是否抛异常,抛什么异常,都使用 fun() throw(); 的方式规范化。
异常总体来说,利大于弊。